InkWell Widget: Flutter has become an increasingly popular framework for building cross-platform mobile applications. One of its standout features is the rich set of widgets it provides for creating intuitive and responsive user interfaces. Among these widgets it is a material widget that responds to touch actions, creating a ripple effect that gives users tactile feedback on their interactions. Understanding the InkWell widget and using it effectively can significantly enhance user experience in your Flutter applications.
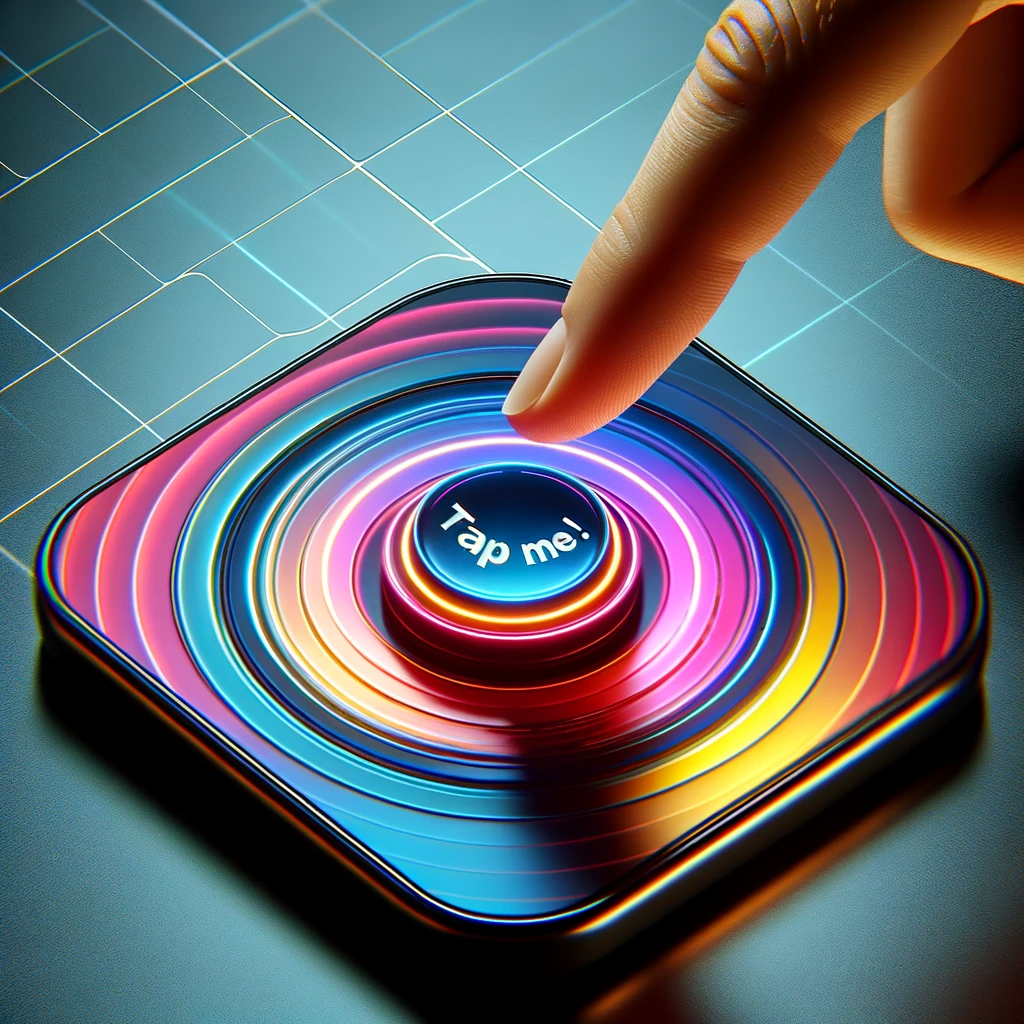
Here’s everything you need to know.
Understanding InkWell
The InkWell
widget wraps an existing widget and adds touch feedback to it. When a user taps the widget, a ripple effect emanates from the point of contact, signaling an interaction. This feedback is crucial in letting users know their touch input has been received and is being processed.
Why Use InkWell?
- Feedback: The InkWell Widget provides a visual indication when users touch an item. This feedback is a key aspect of modern touch interfaces.
- Customization: Flutter allows you to customize the ripple effect, including its color and radius, ensuring it fits well with your app’s design.
- Versatility: It can be used with a variety of widgets, making almost any component interactive.
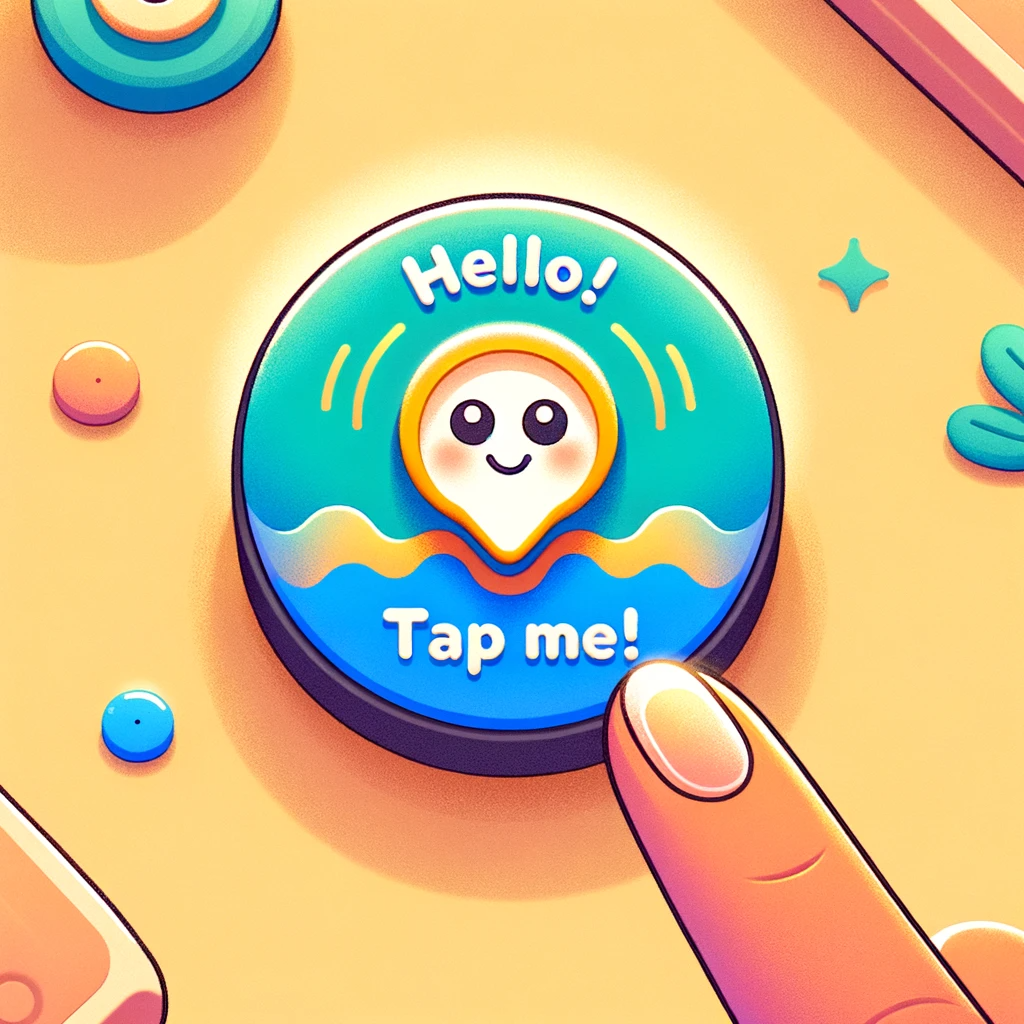
Implementing InkWell Widget
Here’s a simple example of how to implement an InkWell in Flutter:
Widget build(BuildContext context) {
return InkWell(
onTap: () {
// Handle tap.
},
child: Container(
padding: EdgeInsets.all(12.0),
child: Text('Tap me!'),
),
);
}
In this code, the InkWell wraps a container with text. When the container is tapped, the InkWell Widget executes the onTap
callback.
Customizing InkWell Widget
Flutter offers various properties to customize the InkWell’s appearance and behavior:
- onTap, onDoubleTap, onLongPress: Callbacks for different tap actions.
- highlightColor: The color of the overlay appears when any widget is tapped.
- splashColor: The color of the ripple effect.
- radius: The radius of the ripple effect.
Best Practices
- Feedback Consistency: Ensure that all tappable elements in your app provide feedback. Consistency is key to a predictable and comfortable user experience.
- Adequate Targets: Make sure the widgets wrapped by InkWell are large enough to be easily tapped by users. The minimum recommended size is 48×48 pixels.
- Visual Clarity: The ripple effect should be visible against the background of the wrapped widget.
Common Issues and Solutions
- Ink splash not visible: This usually happens when the InkWell is not placed on a Material widget. Wrapping your InkWell with a Material or using an Ink widget can resolve this.
- Overlapping ripples: If multiple InkWell widgets are close to each other, their ripples can overlap. To prevent this, you might need to adjust the radius or the layout.
Conclusion
The InkWell widget is a powerful tool in Flutter for enhancing user interaction. Providing immediate, visual feedback, makes your app feel responsive and intuitive. With the right customization and implementation, you can significantly improve the user experience of your Flutter app.
More Topics:
Mastering the Flutter AppBar: Enhancing User Navigation
ListTile Widget: A Deep Dive into Flutter ListTile Widget
Flexibility: The Flutter Wrap Widget Unwrapped
Mastering the Flutter Row Widget: A Comprehensive Guide
Understanding the Flutter Column Widget: A Comprehensive Guide
Master Flutter ListView Widgets: A Step-by-Step Visual Guide