Flutter ListView: Flutter has become a go-to framework for developing beautiful, natively compiled applications for mobile, web, and desktop from a single codebase. Central to its widget library is the ListView
, an incredibly versatile and essential widget for any Flutter developer. This article will delve into the ListView widget, its uses, and how to effectively implement it in your Flutter applications.
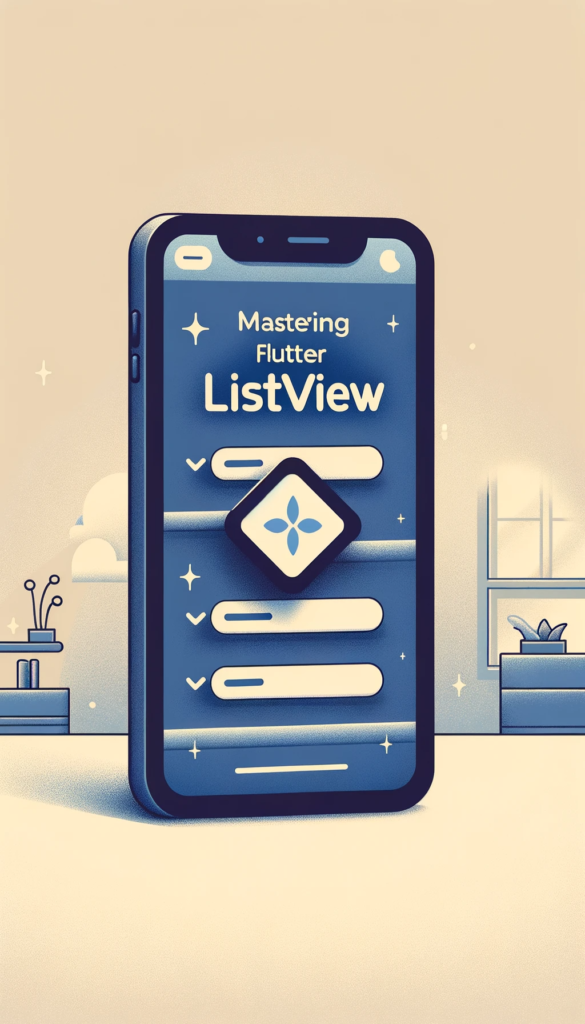
1. What is the ListView Widget?
At its core, the ListView widget allows developers to create a scrollable list of elements. Whether you’re displaying a simple list of text elements or a complex array of widgets, ListView handles it efficiently.
2. Key Features of ListView
ListView is packed with features that make it a must-use widget for developers:
- Scroll Direction: ListView can scroll vertically or horizontally.
- Builder: Use
ListView.builder
for lazy-loading elements, which is memory-efficient. - Separators: Easily add dividers between list items with
ListView.separated
. - Customization: Style your ListView with themes, padding, and more.
3. How to Use ListView in Flutter
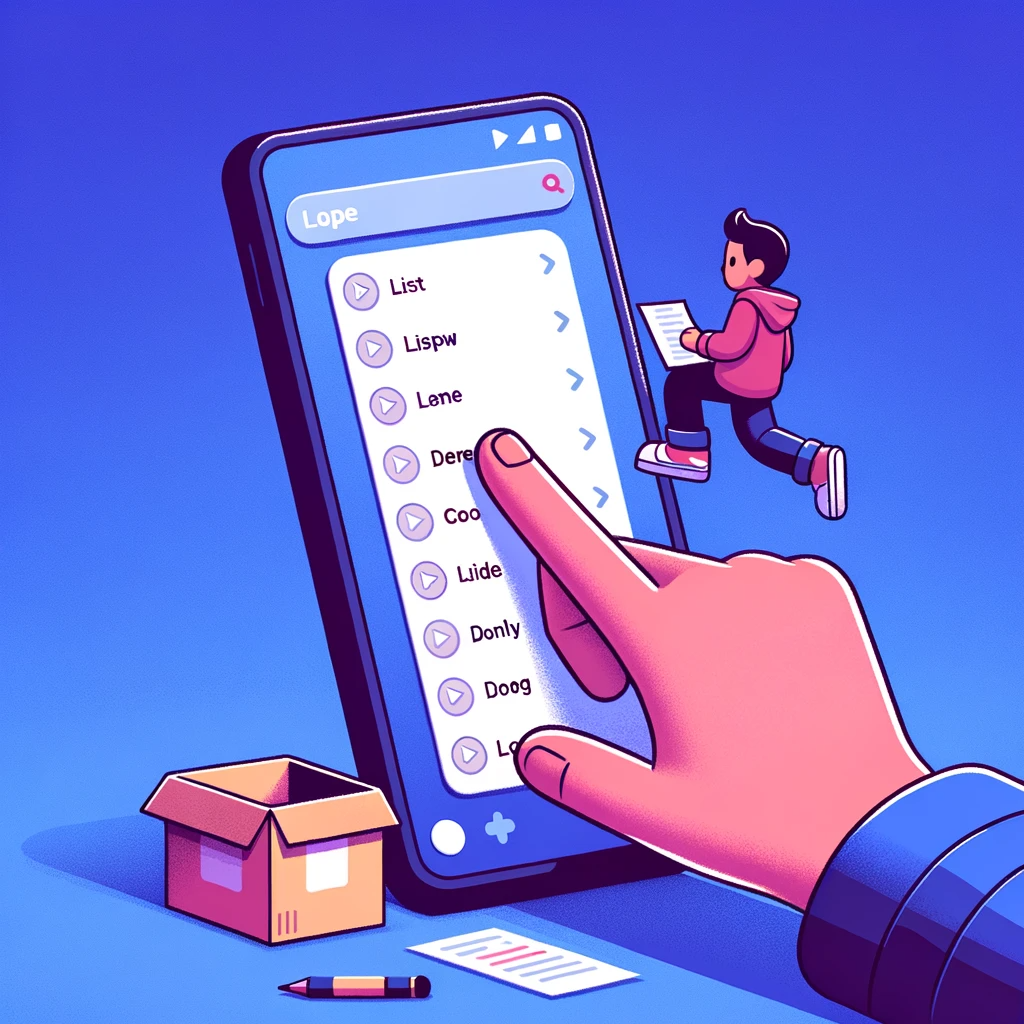
Implementing a ListView in Flutter is straightforward. Here’s a simple example:
ListView(
children: <Widget>[
ListTile(title: Text('Item 1')),
ListTile(title: Text('Item 2')),
ListTile(title: Text('Item 3')),
],
),
ListView.separated
The ListView.separated
constructor creates a list of items with separators in between. It’s perfect for lists where you want a divider between each item.
Here’s an example code snippet:
ListView.separated(
itemCount: 10, // Number of items in the list
separatorBuilder: (context, index) => Divider(color: Colors.grey),
itemBuilder: (context, index) {
return ListTile(
leading: Icon(Icons.star, color: Colors.blueAccent),
title: Text('Item ${index + 1}'),
subtitle: Text('Subtitle for item ${index + 1}'),
trailing: Icon(Icons.arrow_forward_ios),
);
},
)
In this example, ListView.separated
is used to create a list with 10 items, each separated by a grey divider line. Each list item is a ListTile
with a star icon, a title, a subtitle, and a trailing arrow icon.
ListView.builder
The ListView.builder
constructor is ideal for creating a list when you don’t know the number of items upfront or when you want to create an infinite list.
Here’s a simple example:
ListView.builder(
itemCount: 100, // Number of items in the list
itemBuilder: (context, index) {
return ListTile(
title: Text('Dynamic Item ${index + 1}'),
);
},
)
In the ListView.builder
example, the list contains 100 items. The itemBuilder
callback is called only with indices for the items that need to be built, allowing for a more efficient, lazy-loaded list.
4. Customizing Your ListView
Flutter’s ListView is highly customizable. Here’s how you can personalize your lists:
- Themes: Apply global or local themes to change the look of your list items.
- Padding and Margins: Adjust the spacing to suit your design.
- Interactive Elements: Add widgets like switches, checkboxes, or sliders.
5. Best Practices for ListView Performance
To ensure your ListView performs well, especially with large lists:
- Use the Builder:
ListView.builder
only renders items that fit on the screen. - Limit the Use of Dividers: Excessive dividers can lead to performance issues.
- Keep Widgets Light: Opt for simpler widgets within the list for speed and efficiency.
6. Conclusion
The ListView widget is an integral part of Flutter’s widget library, enabling developers to build dynamic and responsive lists with minimal code. By following the best practices outlined in this guide, you can create lists that are not only visually appealing but also performant.
Other Topics:
Flutter: A Deep Dive into the Container Widget with Examples
Flutter Container widget with its all properties | Flutter widgets
Flutter App Bar widget and it’s all properties | Flutter Widgets