In this article, we will learn how can we make a simple flutter counter app with GetX State Management. A counter app is an application in which we can increase or decrease a value by pressing the buttons. An example of this is when we create a flutter application then you see a default flutter application on your screen. So, that is also an example of a counter app.
In this application, we will design a screen in the flutter framework by using the Dart programming language and will increase or decrease the value by initializing the increase and decrease value function inside GetX Controller. In the center, we will show a number that will be updated by pressing the buttons of add or subtract.
Let’s start the work:
First, build a flutter application and write the name of your own choice. Create a dart file with the name of main_screen.dart. Also, create a folder inside the lib folder with the controller name, and inside create a dart file that name will be main_screen_controller.dart. Then call this main_screen in the MyApp.
Files should be like this:
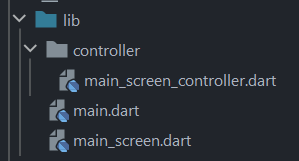
main.dart file code will be like this:
import 'package:counter_app_with_getx/main_screen.dart';
import 'package:flutter/material.dart';
import 'package:get/get.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return GetMaterialApp(
debugShowCheckedModeBanner: false,
title: 'GetX counter app',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MainScreen(),
);
}
}
main_screen_controller.dart file will be:
import 'package:get/get.dart';
class MainScreenController extends GetxController {
RxInt value = 0.obs;
}
Initialize a variable with a value name and make it observable because we need to update this variable value by pressing buttons.
Now, we also write two functions inside GetxController, which we need to update the value of the variable. then its will look like this:
import 'package:get/get.dart';
class MainScreenController extends GetxController {
RxInt value = 0.obs;
void add() {
value.value++;
}
void subtract() {
value.value--;
}
}
main_screen.dart file code will be:
import 'package:counter_app_with_getx/controller/main_screen_controller.dart';
import 'package:flutter/material.dart';
import 'package:get/get.dart';
class MainScreen extends GetView<MainScreenController> {
const MainScreen({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
Get.put(MainScreenController());
return Scaffold(
backgroundColor: Colors.white,
/// app bar
appBar: AppBar(
backgroundColor: Colors.blueAccent,
elevation: 0.0,
centerTitle: true,
title: const Text('Counter App with GetX',
style: TextStyle(
fontSize: 20.0,
color: Colors.white,
),
),
),
/// body
body: SizedBox(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
/// value increase or decrease
Obx(() => Text('Value ${controller.value}',
style: const TextStyle(
fontSize: 60.0,
color: Colors.black,
),
),),
/// buttons to increase or decrease value
const SizedBox(height: 40.0),
Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
FloatingActionButton(
onPressed: (){
controller.add();
},
backgroundColor: Colors.blueAccent,
child: const Center(
child: Icon(Icons.add, color: Colors.white,),
),
),
const SizedBox(width: 40.0),
FloatingActionButton(
onPressed: (){
controller.subtract();
},
backgroundColor: Colors.blueAccent,
child: const Center(
child: Icon(Icons.remove, color: Colors.white,),
),
),
],
),
],
),
),
);
}
}
The output of the flutter counter app
GitHub Link:
https://github.com/flutter99/counter_app_with_getx
Related Articles:
How to make counter app with setState in Flutter
How to make custom gradient buttons in Flutter
Flutter UI, Splash Screen, Login, Signup and Forgot Password
1 Response
[…] How to make Flutter Counter App with GetX […]