In this article, we will learn how can we make a simple flutter counter app with setState. A counter app is an application in which we can increase or decrease a value by pressing the buttons. An example of this is when we create a flutter application then you see a default flutter application on your screen. So, that is also an example of a counter app.
In this application, we will design a screen in the flutter framework by using the dart programming language. In the center, we will show a number that will be updated by pressing the buttons of add or subtract.
Let’s start the work:
First, build a flutter application and write the name of your own choice. Create a dart file with the name of main_screen.dart. Then call this main_screen in the MyApp.
Files should be like this:
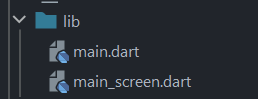
main.dart file code will be like this:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Counter App',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MainScreen(),
);
}
}
Now we create the design on the main_screen.dart file. First I will create an app bar in which I write the title Counter App and code will be:
/// appbar
appBar: AppBar(
backgroundColor: Colors.black,
elevation: 0.0,
centerTitle: true,
title: const Text('Counter App',
style: TextStyle(
fontSize: 24.0,
color: Colors.white,
),
),
),
In the body, will add two floating actions buttons in a row.
Row( crossAxisAlignment: CrossAxisAlignment.center, mainAxisAlignment: MainAxisAlignment.center, children: [ FloatingActionButton( onPressed: (){ }, backgroundColor: Colors.black, child: const Icon(Icons.add, color: Colors.white,), ), SizedBox(width: 80.0), FloatingActionButton( onPressed: (){ }, backgroundColor: Colors.black, child: const Icon(Icons.remove, color: Colors.white,), ), ], ),
Now I will write two methods to increase and decrease the value and that should add() and subtract()
void add() {
setState((){
value ++;
});
}
void subtract() {
setState((){
value --;
});
}
Source code:
//// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Counter App',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MainScreen(),
);
}
}
/// main_screen.dart
import 'package:flutter/material.dart';
class MainScreen extends StatefulWidget {
const MainScreen({Key? key}) : super(key: key);
@override
State<MainScreen> createState() => _MainScreenState();
}
class _MainScreenState extends State<MainScreen> {
int value = 0;
void add() {
setState((){
value ++;
});
}
void subtract() {
setState((){
value --;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.white,
/// appbar
appBar: AppBar(
backgroundColor: Colors.black,
elevation: 0.0,
centerTitle: true,
title: const Text('Counter App',
style: TextStyle(
fontSize: 24.0,
color: Colors.white,
),
),
),
/// body
body: SizedBox(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('Value $value',
style: const TextStyle(
fontSize: 60.0,
color: Colors.black,
),
),
SizedBox(height: 40.0),
Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
FloatingActionButton(
onPressed: (){
add();
},
backgroundColor: Colors.black,
child: const Icon(Icons.add, color: Colors.white,),
),
SizedBox(width: 80.0),
FloatingActionButton(
onPressed: (){
subtract();
},
backgroundColor: Colors.black,
child: const Icon(Icons.remove, color: Colors.white,),
),
],
),
],
),
),
);
}
}
The output of the flutter counter app
Source Code:
https://www.github.com/flutter99/counter_app
Related Articles:
Flutter UI, Splash Login Signup Forgot Password Screen
2 Responses
[…] How to make counter app with setState in Flutter […]
[…] How to make counter app with setState in Flutter […]