In this article, we will learn how we can make the gradient icon in Flutter.
What is Flutter Framework?
Flutter is Google’s portable UI toolkit for crafting beautiful, natively compiled applications for mobile, web, and desktop from a single codebase. Flutter works with existing code, is used by developers and organizations around the world, and is free and open source.
Let’s the problem is here that, there are many apps that have gradient colors icons. But in Flutter there is no gradient method on the Icon widget. So, that’s why we will be using the ShaderMask Widget to make the gradient color of icons. We need to wrap the icon with this ShaderMask Widget. Let’s make a custom class with the GradientIcon name.
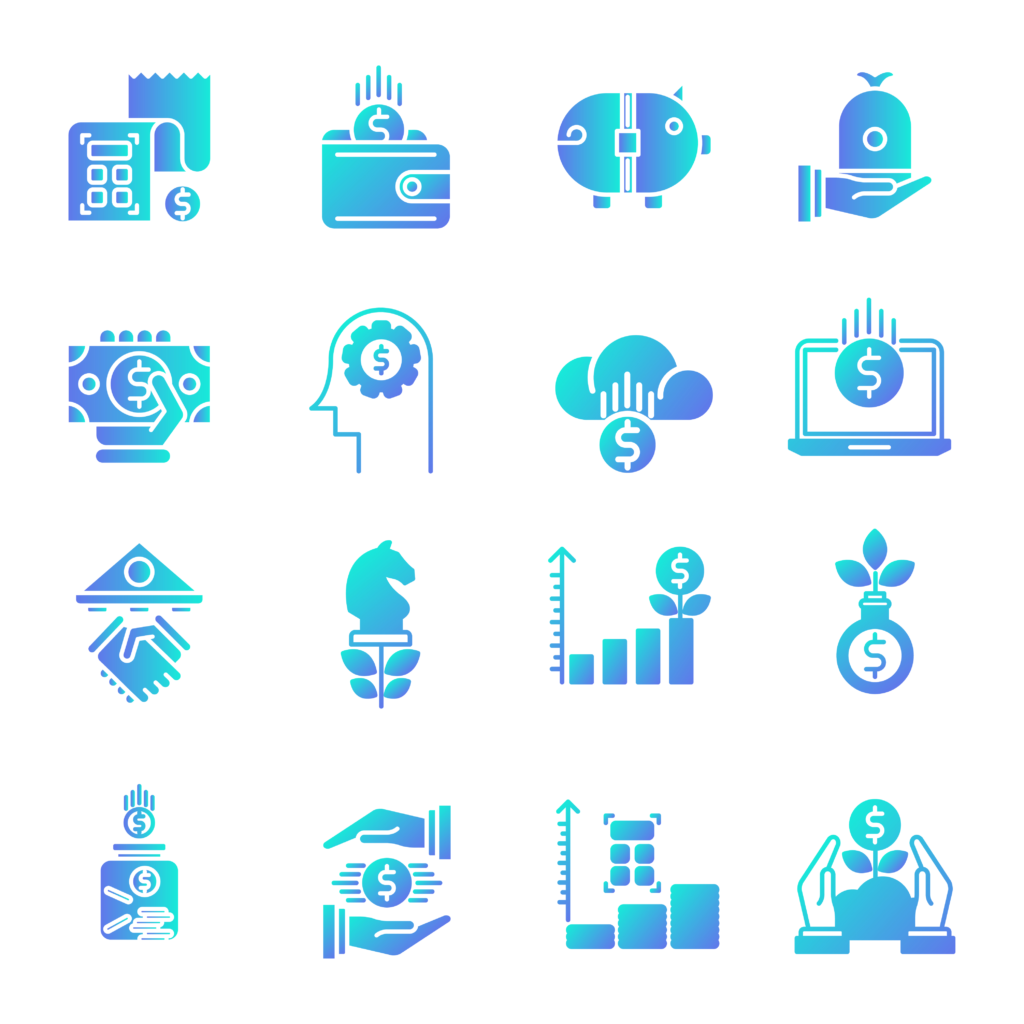
Here is the class for the GradientIcon widget:
class GradientIcon extends StatelessWidget {
GradientIcon(
this.icon,
this.size,
this.gradient,
);
final IconData icon;
final double size;
final Gradient gradient;
@override
Widget build(BuildContext context) {
return ShaderMask(
child: SizedBox(
width: size * 1.2,
height: size * 1.2,
child: Icon(
icon,
size: size,
color: Colors.white,
),
),
shaderCallback: (Rect bounds) {
final Rect rect = Rect.fromLTRB(0, 0, size, size);
return gradient.createShader(rect);
},
);
}
}
Read this also: How to Call SetState in Dialog Flutter
In order to use this, all you need to do is to pass the icon and gradient that you would like to apply:
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'package:new_page/screens/custom_gradient_icon.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const GetMaterialApp(
debugShowCheckedModeBanner: false,
title: 'Custom Gradient Icon',
home: CustomGradientIcon(),
);
}
}
import 'package:flutter/material.dart';
import 'package:new_page/widgets/gradient_icon.dart';
class CustomGradientIcon extends StatelessWidget {
const CustomGradientIcon({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
double height = MediaQuery.of(context).size.height;
double width = MediaQuery.of(context).size.width;
return Scaffold(
backgroundColor: Colors.white,
body: SizedBox(
height: height,
width: width,
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
GradientIcon(
Icons.keyboard,
80.0,
const LinearGradient(
colors: [
Colors.yellow,
Colors.purple,
],
begin: Alignment.topLeft,
end: Alignment.centerRight,
),
),
],
),
),
);
}
}
Read this also: Custom Splash Screen Widget
Gradient Icon in Flutter
In this way, you can make the Gradient Icon in Flutter
Gradient icon in flutter
2 Responses
[…] How to make gradient icon in Flutter 2022 […]
[…] Flutter UI Gradient Icon […]