In this topic, we will discuss how you can create a custom widget for a splash screen on flutter. So, to move on to our topic first, we will discuss the custom widget.
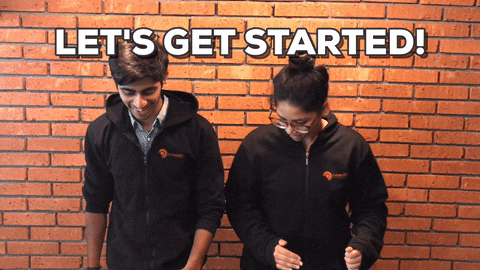
What is Custom Widget?
In Flutter, we can say that a custom widget is a custom shape that we need to our requirements. We declare all the parameters in the custom widget class according to our requirements, create a constructor for them, and use it anywhere in the whole app where we need it.
Let’s show an example of a custom button:
class CustomButton extends StatelessWidget {
final VoidCallback onTap;
final double? btnHeight;
final double? btnWidth;
final Color? btnColor;
final String? btnText;
final Color? btnTextColor;
const CustomButton({Key? key,
required this.onTap,
this.btnHeight = 50.0,
this.btnWidth = 350.0,
this.btnColor = Colors.blue,
this.btnText = 'Button',
required this.btnTextColor,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: onTap,
child: Container(
height: btnHeight,
width: btnWidth,
decoration: BoxDecoration(
color: btnColor,
borderRadius: BorderRadius.circular(8.0),
),
child: Center(
child: Text(btnText!,
style: TextStyle(
fontSize: 18.0,
color: btnTextColor,
fontWeight: FontWeight.bold,
),
),
),
),
);
}
}
Here is an example of the custom widget on which we create a simple custom button for our flutter app. This custom button we can use in the whole app with our custom shape of the button.
See this Also: Make Transparent Appbar In Flutter
Splash Screen Custom Widget:
To make a custom widget for a splash screen, we will declare all parameters in this way, So, we can use them by our own requirements. You can save this custom widget into your git repository and use it again anytime for your next project without applying effort again on it.
First, we create a stateless class with SplashScreen name.
class SplashScreen extends StatelessWidget {
const SplashScreen({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container();
}
}
Now we make it a proper custom widget and declare all the parameters that are necessary for us in every splash screen.
If the logo of the app is in the SVG format then we need to add a package in pubspec.yaml file, that name is flutter_svg.
dependencies:
flutter_svg: ^1.0.1
Otherwise, we can use Image.asset widget.
class SplashScreen extends StatelessWidget {
final Color? backgroundColor;
final double? iconHeight;
final double? iconWidth;
final bool? isSvg;
final String? iconPath;
final VoidCallback onPressed;
SplashScreen({Key? key,
required this.isSvg,
this.backgroundColor = Colors.white,
this.iconHeight = 80.0,
this.iconWidth = 80.0,
required this.iconPath,
required this.onPressed,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: onPressed,
child: Container(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
color: backgroundColor,
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
SizedBox(
height: iconHeight,
width: iconWidth,
child: isSvg == true ? SvgPicture.asset(iconPath!) : Image.asset(iconPath!,
fit: BoxFit.fill,
),
),
],
),
),
);
}
}
Here is the Custom widget for the splash screen. You can add more parameters also according to your own requirements. You just need to follow the structure of the code and make your own custom widgets for the whole app.
Complete Code:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Custom Widget',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: SplashScreen(
isSvg: false,
iconPath: 'assets/bg.jpg',
onPressed: (){},
),
);
}
}
class SplashScreen extends StatelessWidget {
final Color? backgroundColor;
final double? iconHeight;
final double? iconWidth;
final bool? isSvg;
final String? iconPath;
final VoidCallback onPressed;
SplashScreen({Key? key,
required this.isSvg,
this.backgroundColor = Colors.white,
this.iconHeight = 80.0,
this.iconWidth = 80.0,
required this.iconPath,
required this.onPressed,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: onPressed,
child: Container(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
color: backgroundColor,
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
SizedBox(
height: iconHeight,
width: iconWidth,
child: isSvg == true ? SvgPicture.asset(iconPath!) : Image.asset(iconPath!,
fit: BoxFit.fill,
),
),
],
),
),
);
}
}
Read this article: Top Highest Paid Skills on Fiverr (2022)
Another article: Top 5 fast-growing programming languages
In this way, you can make a custom widget and also can use this custom splash screen widget.
For more interesting blogs, skills articles, programming articles you can follow us. Thanks
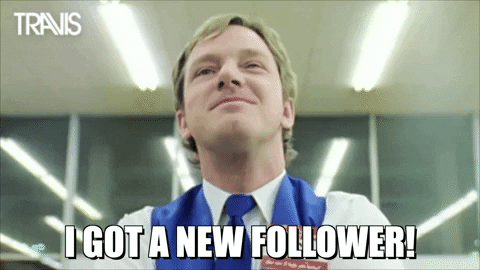
2 Responses
[…] Flutter – Splash Screen Custom Widget […]
[…] Read this also: Custom Splash Screen Widget […]