In this article, we will learn how a Flutter developer can make a Transparent App bar for an app or web app.
Making the Transparent App Bar is quite easy. So, let’s start from scratch.
- Go to the Android Studio or VS Code and create a New Flutter Project.

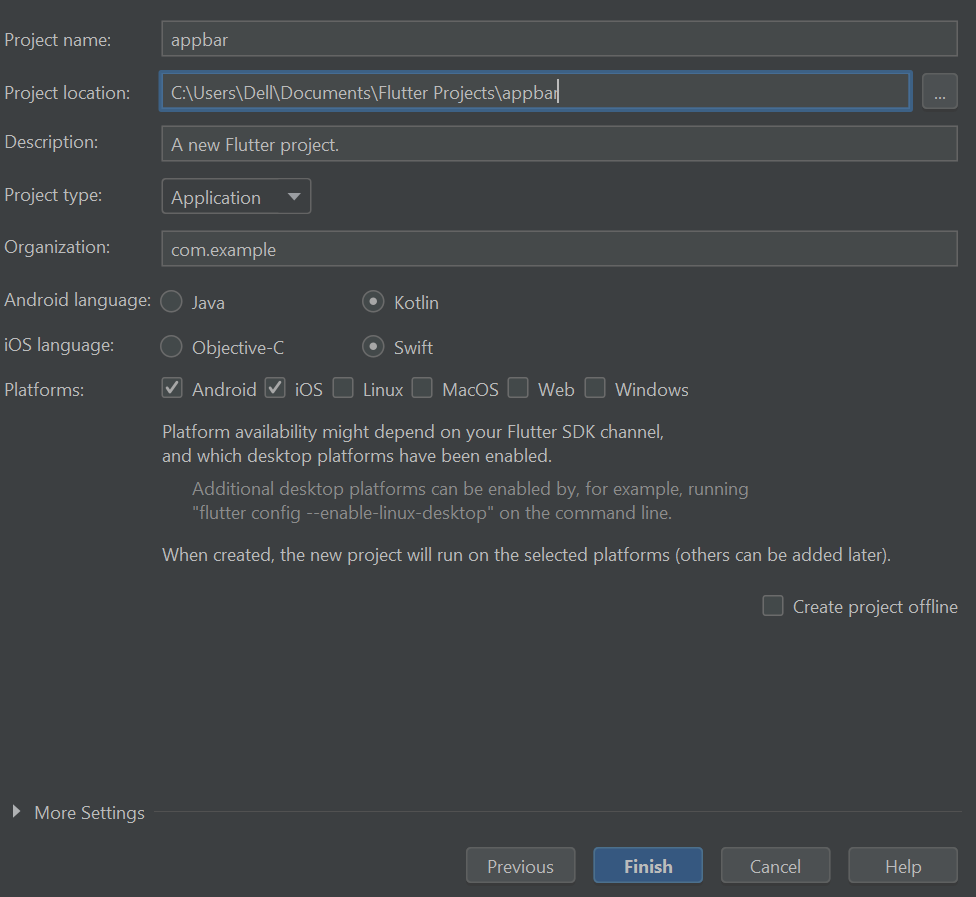
2. Create a Stateless or Stateful widget and the name of the widget AppBar
class Appbar extends StatelessWidget {
const Appbar({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container();
}
}
3. Under this Appbar class return the Scaffold widget and call app bar.
class Appbar extends StatelessWidget {
const Appbar({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Transparent Appbar'),
centerTitle: true,
),
);
}
}
4. Now run the code by clicking the run button that is in green color.
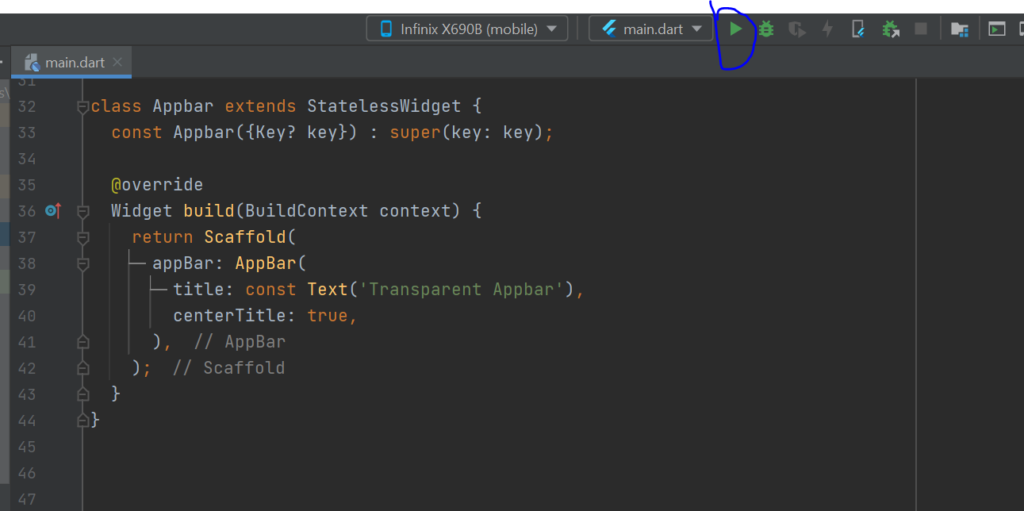
By default, you will see the app bar color like this.
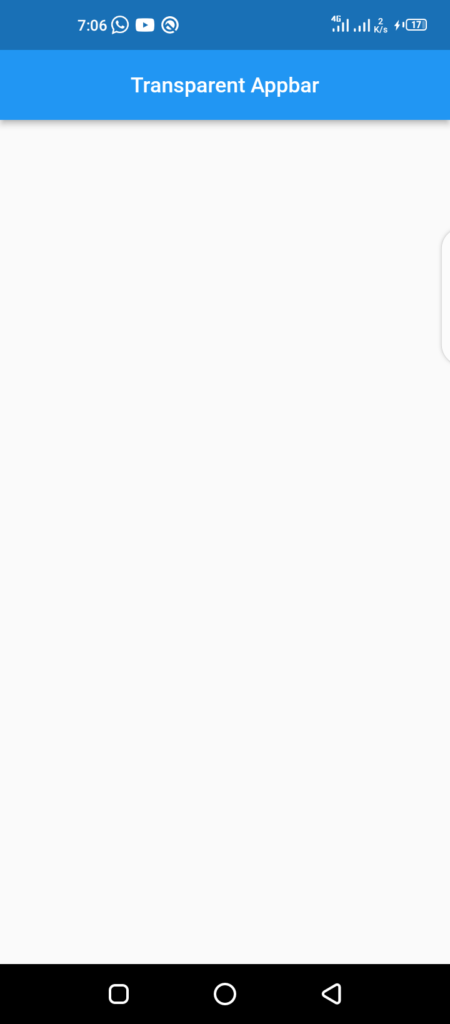
5. Now we put the image in the background. To put the background image we create a new stateless widget with the ‘BgImage’ name. You can write code like this
class BgImage extends StatelessWidget {
const BgImage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return SizedBox(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
child: Image.asset('assets/bg.jpg',
fit: BoxFit.fill,
),
);
}
}
and the App bar stateless code will be like this
class Appbar extends StatelessWidget {
const Appbar({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Stack(
children: [
const BgImage(),
Scaffold(
backgroundColor: Colors.transparent,
appBar: AppBar(
title: const Text('Transparent Appbar'),
centerTitle: true,
),
),
],
);
}
}
Then the output should be like this.
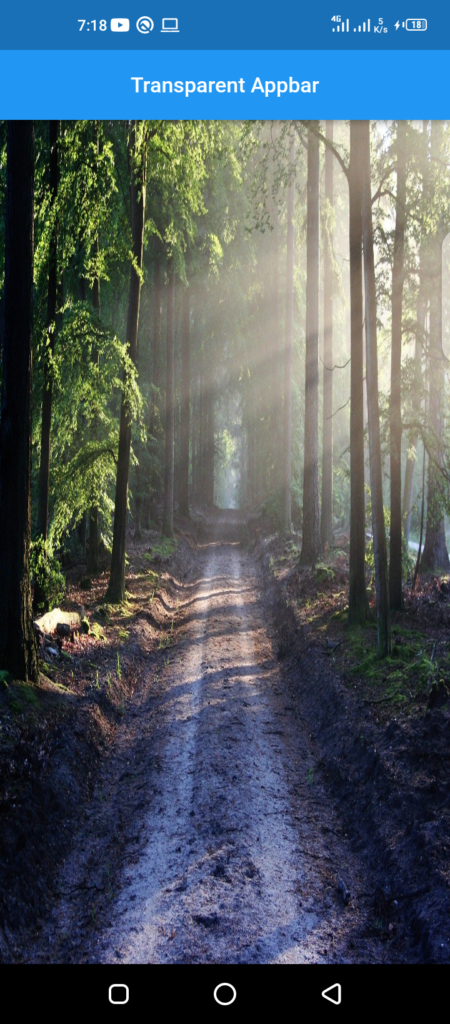
6. To make the app bar color transparent we need to set the background transparent color of the app bar and also need to set elevation 0.
class Appbar extends StatelessWidget {
const Appbar({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Stack(
children: [
const BgImage(),
Scaffold(
backgroundColor: Colors.transparent,
appBar: AppBar(
title: const Text('Transparent Appbar',
style: TextStyle(color: Colors.white, fontSize: 24.0),
),
centerTitle: true,
backgroundColor: Colors.transparent,
elevation: 0,
),
),
],
);
}
}
The output will be with an app bar transparent color.
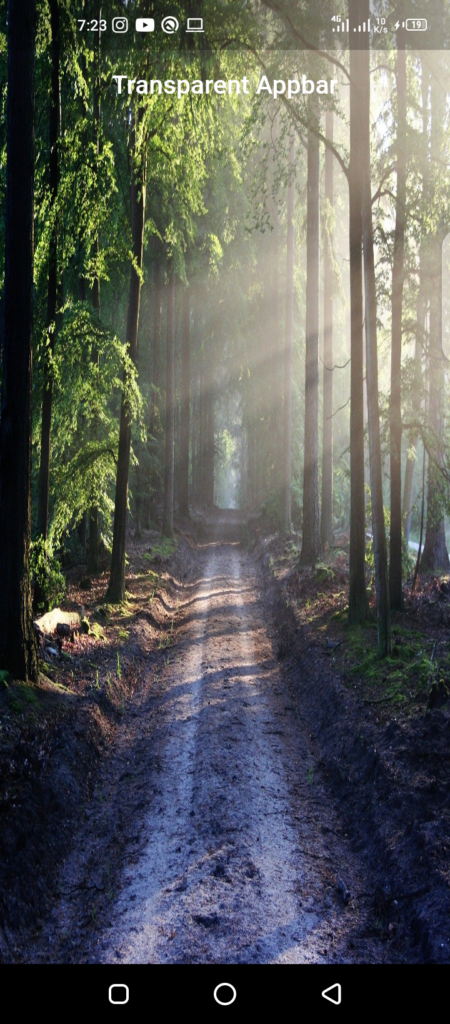
Complete Code of Transparent App Bar:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const Appbar(),
);
}
}
class Appbar extends StatelessWidget {
const Appbar({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Stack(
children: [
const BgImage(),
Scaffold(
backgroundColor: Colors.transparent,
appBar: AppBar(
title: const Text('Transparent Appbar',
style: TextStyle(color: Colors.white, fontSize: 24.0),
),
centerTitle: true,
backgroundColor: Colors.transparent,
elevation: 0,
),
),
],
);
}
}
class BgImage extends StatelessWidget {
const BgImage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return SizedBox(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
child: Image.asset('assets/bg.jpg',
fit: BoxFit.fill,
),
);
}
}
Here is the best way to make the transparent color of appbar in flutter.
#Flutter #transparent #background #appbar
If you have any questions about the Flutter UI work you can write a comment here. We are always here to help you.
Read this Article: Top highest paid skills on Fiverr 2022
Transparent App Bar Color in Flutter 2022
Flutter UI Work
4 Responses
[…] See this Also: Make Transparent Appbar In Flutter […]
[…] How to make a transparent appbar in Flutter […]
[…] Read this article: How to make transparent appbar in Flutter […]
[…] Read this article: How to make transparent appbar in Flutter […]